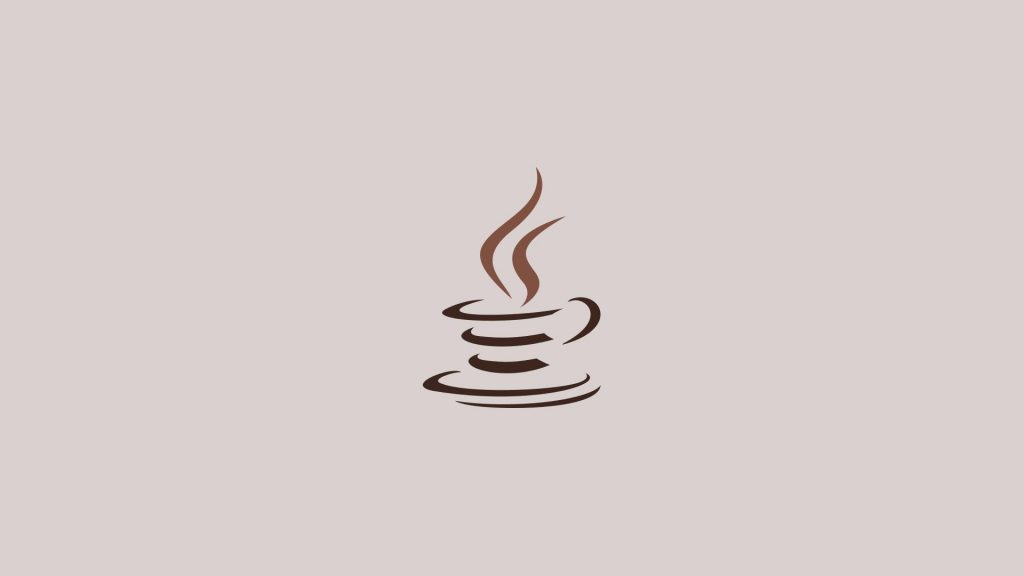
Java: Geometry: great circle distance
package chapter4_4_2; import java.util.Scanner; /** * * @author Siavash Bakhshi */ public class Chapter4_4_2 { /** * @param args the command line arguments */ public static void main(String[] args) { // Add Scanner to read input from user Scanner input = new Scanner(System.in); // text to prompt to user asking to enter a number System.out.print("Enter point 1 (latitude and longitude) in degrees: "); String xy1in = input.nextLine(); System.out.print("Enter point 2 (latitude and longitude) in degrees: "); String xy2in = input.nextLine(); String[] xy1 = xy1in.split(","); String[] xy2 = xy2in.split(","); //Declare Parameters double radius = 6371.01; double x1 = Math.toRadians(Double.parseDouble(xy1[0])); double y1 = Math.toRadians(Double.parseDouble(xy1[1])); double x2 = Math.toRadians(Double.parseDouble(xy2[0])); double y2 = Math.toRadians(Double.parseDouble(xy2[1])); //The great circle distance formula: double d = radius * Math.acos((Math.sin(x1) * Math.sin(x2)) + (Math.cos(x1) * Math.cos(x2)) * (Math.cos(y1 - y2))); System.out.println("The distance between the two points is " + d + "km"); } }Java: Geometry: great circle distance (625 downloads)